Overlap Elements With CSS Grid Instead Of Position Absolute
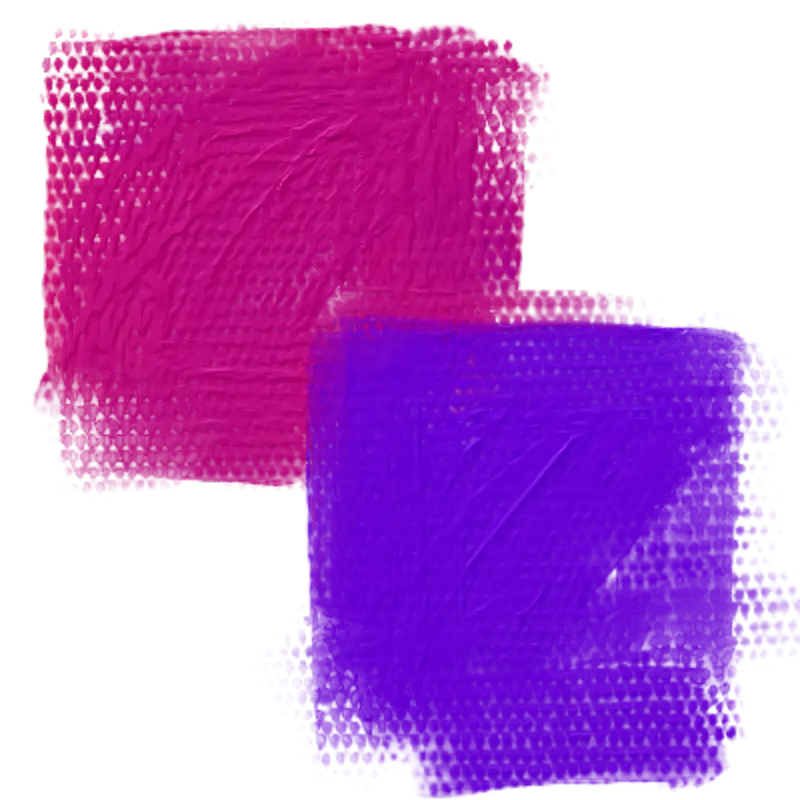
We can use CSS absolute positioning to overlap elements, but there’s some advantages to using CSS grid instead. Let’s explore using CSS grid as an alternative to position absolute.
Overlapping Elements With Position Absolute
Using the styles below we can position multiple child elements relative to the first parent element that has a position
property, in this case the direct parent.
<div class="parent">
<div class="child">Robert</div>
<div class="child">Charlene</div>
<div class="child">Baby</div>
</div>
<style>
.parent {
position: relative;
}
.child {
position: absolute;
top: 0;
left: 0;
}
</style>
Below we can see absolute
positioning in action, the different children names are rendered on top of each other.
Right. That looks objectively horrible.
Now let’s try to achieve the same result with CSS Grid.
Overlapping Elements With Grid
We set display
to grid
on the parent element, and we define one grid column. 1fr
means 1 part of all the available space, which in this case is everything.
We instruct the children to all occupy this one grid column. ⛺️
<div class="parent">
<div class="child">Robert</div>
<div class="child">Charlene</div>
<div class="child">Baby</div>
</div>
<style>
.parent {
display: grid;
grid-template-columns: 1fr;
}
.child {
grid-row-start: 1;
grid-column-start: 1;
}
</style>
Let’s check out the equally horrible result below.
It looks the same, but there’s an important difference.
Where the children in the absolute positioning example no longer affect page flow, meaning they no longer take up any vertical space, the children in the grid example do.
The parent in the positioning example has zero height where the height of the parent in the grid example equals the max child height.
Let’s look at them together, the position absolute example has a red outline, the grid example a green one.
So why is this relevant?
This is very useful when we need to animate overlapping elements but want to retain normal page flow.
Imagine a tab control where tab panels crossfade. With absolute positioning we would have to fix the height of the tab control. With grid the height is automatically derived from the child elements.
Conclusion
Absolute positioning has historically been the only way to effectively overlap elements. Unfortunately this meant detaching elements from the document layout, forcing us to assign a fixed or minimum height.
Using Grid we can overlap elements while retaining height resulting in more stable and clean code.